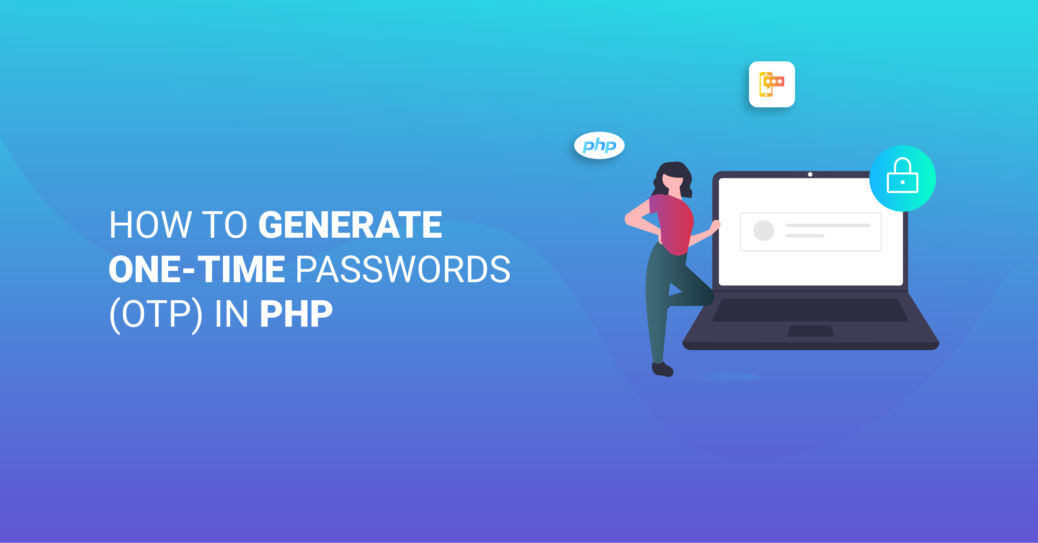
How to generate one time password (OTP) in PHP
Generate one time password (OTPCode) in PHP
To generate a one time password (OTP) in PHP, you can use a library like Google’s PHP-OTP library or write your own code to generate OTPs using a secure random number generator and an OTP algorithm like TOTP
(Time-based One-Time Password) or HOTP
(HMAC-based One-Time password).
Here’s an example of how you could generate an TOTP
OTP
in PHP:
<?php // Use the hash_hmac function to generate the OTP function generateOTP($secret, $time, $length = 6) { // The OTP is a 6-digit code, so we use 6 zeros as the pad $paddedTime = str_pad($time, 16, '0', STR_PAD_LEFT); // Use the SHA1 algorithm to generate the OTP $hash = hash_hmac('sha1', $paddedTime, $secret, true); // Extract the 4 least significant bits of the last byte of the hash $offset = ord(substr($hash, -1)) & 0xF; // Extract a 4-byte string from the hash starting at the $offset $truncatedHash = substr($hash, $offset, 4); // Convert the 4-byte string to an integer $code = unpack('N', $truncatedHash)[1] & 0x7FFFFFFF; // Return the OTP as a 6-digit code return str_pad($code % 1000000, $length, '0', STR_PAD_LEFT); } // Set the secret key for the OTP $secret = 'JBSWY3DPEHPK3PXP'; // Set the current time as the $time parameter $time = time(); // Generate the OTP $otp = generateOTP($secret, $time); echo $otp;
This code will generate a TOTP
OTP
based on the current time and the secret key provided. To verify the OTP
, you can use the same function with a slightly different secret key and time value and compare the generated OTP
to the one received from the user.
You can customize the function to use a different length for the OTPs by modifying the $length
parameters.
Note that it’s important to use a secure random number generator to generate the secret key, and to store it securely. You should also consider using a more secure hashing algorithm than SHA1
for generating the OTP
.